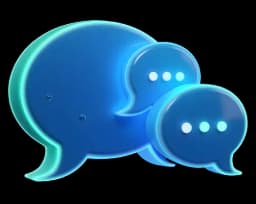
No Comments Yet
Be the first to share your thoughts and start the conversation.
Be the first to share your thoughts and start the conversation.
As I said, it’s not related to Next.js. You can do it in React.js too. But preferring URL as state management in Next.js will solve 90% of your problems and save you from making everything client-side
So how do we do that?
Think, what happened when we visited Amazon. We typed something, hit enter, and the same thing appeared in the URL. Do the same for filters. Select something, and it appears in the URL.
Got it?
Yeah, so all we have to do is, whenever a user types something or selects something, we add that selected or typed info in the URL.
There are many ways. Let’s see how we can do that one by one using Next.js
Next.js Link
It’s straightforward. For example,
<Link
href={{
pathname: "/jobs",
query: { type: "softwaredeveloper" },
}}
>
All Jobs
</Link>
And doing this will result in
Whatever your query is, you specify it inside the , and whenever someone clicks on it, it’ll form the right URL with that corresponding query
Next.js Router
You can use the Next.js hook and route to any page with a query like this
"use client";
import { useRouter } from "next/navigation";
const MyComponent = () => {
const router = useRouter();
const handleButtonClick = () => {
router.push({
pathname: "/search",
query: { q: "your_search_query_here" },
});
};
return <button onClick={handleButtonClick}>Search</button>;
};
export default MyComponent;
And doing this will result in
💡 Yes, you’re right. Using will turn your page/component into a client page/component. So be careful when you want to use it
Programmatically
We can use a built-in JavaScript object, , to create a new URL and navigate to it using or
Here is a short sweet example of that,
const handleButtonClick = () => {
const searchParams = new URLSearchParams(window.location.search);
searchParams.set("q", "your_search_query_here");
window.location.href = `${window.location.pathname}?${searchParams.toString()}`;
};
Learn more about here:
For a few of you, this might feel a bit too much. But this can come super handy when we’ve to change URL parameters frequently on user actions. For example, when doing a filter. Here is what it would look like
export const updateSearchParams = (type: string, value: string) => {
// Get the current URL search params
const searchParams = new URLSearchParams(window.location.search);
// Set the specified search parameter to the given value
searchParams.set(type, value);
// Set the specified search parameter to the given value
const newPathname = `${window.location.pathname}?${searchParams.toString()}`;
return newPathname;
};
export const deleteSearchParams = (type: string) => {
// Set the specified search parameter to the given value
const newSearchParams = new URLSearchParams(window.location.search);
// Delete the specified search parameter
newSearchParams.delete(type.toLocaleLowerCase());
// Construct the updated URL pathname with the deleted search parameter
const newPathname = `${
window.location.pathname
}?${newSearchParams.toString()}`;
return newPathname;
};
NPM Package
Yeah yeah, we have a library for that as well. And the one that I like and personally recommend is query-string.
But why bother using it?
The above solutions will work perfectly fine if it’s a small application where you don’t have too many things to handle in the URL.
However, as the application becomes complex, it gets a bit difficult to manage all parameters in the URL.
For example, if your application might have search, filter, and pagination all in one place. And if the user wants to search for something with a filter and pagination enabled, you have to form a query in that way, i.e.,
We have to make sure that typing something in the search box doesn’t reset the parameters already present in the URL. Same with filters or pagination. Users should be able to add more parameters with ease.
This is called preserving URL history/state so we don’t lose the previous information and provide the best UX
To effectively manage this, I recommend using . This is how you can create URLs while preserving their previous version,
export function formUrlQuery({ params, key, value }: UrlQueryParams) {
const currentUrl = qs.parse(params);
currentUrl[key] = value;
return qs.stringifyUrl(
{
url: window.location.pathname,
query: currentUrl,
},
{ skipNull: true },
);
}
You can call wherever you want, like this,
/
const handleUpdateParams = (value: string) => {
const newUrl = formUrlQuery({
params: searchParams.toString(),
key: "location",
value,
});
router.push(newUrl);
};
Sure, there might be more options for achieving the same thing. So as I said, choose the ones that work best for you & your problem case.
But that’s how we do URL as state management in Next.js or even React.js. I hope you understand it!
How did you manage to remove the blur property and reach here?
Upgrading gives you access to quizzes so you can test your knowledge, track progress, and improve your skills.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we discuss the effective approach of using URL state management in Next.js and React to enhance user interactions and improve state handling. Implementing state management through the URL can simplify problem-solving in applications by making state changes visible in the URL itself, mimicking patterns used in large applications like Amazon.
useRouter
hook in Next.js allows for programmatic URL changes from client pages, though it may render the page client-side.00:00:02 So by now you hopefully understand why did we decide to use the simplest and yet the most effective approach of state management in Next.js,
00:00:11 URL state management.
00:00:13 But now, let's talk a bit about how you can do it effectively.
00:00:17 As I said, it's not related to Next.js.
00:00:19 You can do it in React, too.
00:00:21 But preferring URL as the state management solution in Next.js will solve 90% of your problems and save you from making everything client-side.
00:00:30 So, how do we do it?
00:00:31 Think about Amazon.
00:00:33 When we typed something into a search bar or an input, hit Enter, and the same thing appeared in the URL.
00:00:40 Same thing for the filters.
00:00:42 Click something, the checkbox takes on the website, and it appears in the URL.
00:00:46 Do you get it?
00:00:47 So all we have to do is whenever a user types something or selects something, we have to add that or type it out in the URL.
00:00:56 And there are many ways of doing it.
00:00:57 The first one is through a Next.js link.
00:01:00 Simple and straightforward.
00:01:02 The link actually changes the URL, like the path name and even the query.
00:01:07 By doing this, your URL will look like this, forward slash jobs, question mark type is equal to software developer.
00:01:15 Whatever your query is, you specify it inside the href, and whenever someone clicks on it, it'll form the right URL with the right query.
00:01:24 The second way is using a Next.js use router hook to do it within client pages.
00:01:30 There, you can use router.push and do the same thing as before.
00:01:34 Doing this will result in the same thing.
00:01:36 But again, you're right, this will turn it into a client page, so be careful when you use it.
00:01:41 Another way is to do it programmatically by using built-in JavaScript objects, such as URL search programs, to create a new URL and navigate to it using
00:01:53 useRouter or WindowLocation.
00:01:54 A simple example is, again, creating your own search params, setting the query, and then re-navigating your browser to that location.
00:02:02 I hope this isn't too much, but it can come super handy when we have to change the URL parameters frequently on any user action,
00:02:11 for example, when doing a filter.
00:02:13 And here is how that would look like in a real example.
00:02:17 I have created a utility function called updateSearchParams, which takes in the type of the param to update and its value.
00:02:25 First, we construct the URL params object.
00:02:29 We set the specified search parameter, and then we append it to the URL that we have right now, just the path name.
00:02:36 In the same way, once somebody unchecks that checkbox or deletes their search bar, we have to also focus on deleting it from the URL bar.
00:02:44 That's it.
00:02:45 And of course, whenever you have a lot of code that people often have to type, there's a library for that.
00:02:50 So in this case, there's a query string library, which I like using, which simplifies things significantly.
00:02:57 You can create a new utility function called form URL query, which takes in the current URL, and then you can update it with the key and the value.
00:03:07 And finally, you can return the updated URL, which you can then call like this const new URL is equal to form URL query with the following params,
00:03:17 the key, and the value.
00:03:19 And then you finally push to it.
00:03:21 Look, I know that this isn't simple, especially when I laid out so much information about state management in XGIS in a couple of these text-based lessons.
00:03:32 But moving forward in this course, we'll actually do it all within our Devflow project.
00:03:38 So you'll be able to learn and practice exactly what we covered right here.
00:03:43 Think of these lessons just as an introduction.
00:03:45 But now that you have the general information and the why we're doing it, learning how to do it will be simple.