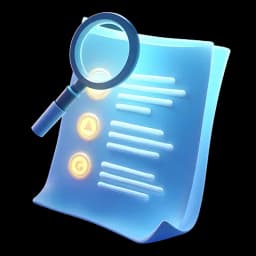
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
Before we get started with understanding how we can do URL state management in Next.js, let’s see how we can get various URL info in Next.js
A URL (Uniform Resource Locator) with parameters typically consists of several components:
Scheme: Specifies the protocol used to access the resource, such as or .
Domain: The domain name or IP address of the server hosting the resource.
Port: (Optional) Specifies the port number to which the request should be sent. Default ports are often omitted (e.g., port for HTTP, port for HTTPS).
Path: The specific resource or endpoint on the server, typically represented as a series of directories and filenames.
Query Parameters: (Also known as in Next.js) Additional data sent to the server as part of the request, typically used for filtering or modifying the requested resource. Query parameters are appended to the URL after a question mark and separated by ampersands
For example:
Fragment: (Optional) Specifies a specific section within the requested resource, often used in web pages to navigate to a particular section. It is indicated by a hash followed by the fragment identifier.
We can retrieve this URL information in Next.js in different ways:
Page If you’re on the main file, then you can access the information through props
function Page({ params, searchParams }) {
return <h1>My Page</h1>;
}
export default Page;
For example, If the URL looks like this:
Then,
async function Page({ params, searchParams }) {
const { id } = await params;
const { page, filter } = await searchParmas;
return <h1>My Page</h1>;
}
export default Page;
💡 You can learn more about and here
From here, you can choose to pass these values to other components or use other options to access them specifically inside that component And that other way is,
Hooks Next.js provides two specific hooks, namely and , to retrieve the respective information from the URL This is how we can access (dynamic part of the URL)
"use client";
import { useParams } from "next/navigation";
function ExampleClientComponent() {
const params = useParams();
return <p>Example Client Component</p>;
}
export default ExampleClientComponent;
You can learn more about hook here. We can access searchParams (aka query parameters) of URL in the same way, using the hook
"use client";
import { useSearchParams } from "next/navigation";
export default function SearchBar() {
const searchParams = useSearchParams();
const type = searchParams.get("type");
return <>Selected Type: {type}</>;
}
Learn more about the hook here.
Yes, you’re right. Using hooks would mean turning that component into a client component.
As a rule of thumb, if your component is near its parent , then instead of opting for these hooks, you can pass and of props to its respective children. A bit of prop drilling won’t hurt.
But if the component is far away from , you should use the above-mentioned hooks instead.
Now that you know how to access information from the URL, let’s see how we can add any kind of information to the URL in Next.js
See you in the next lesson 👋
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:02 Before we dive deeper into understanding how to do URL state management in Next.js, let me teach you how you can get different parts of the URL information
00:00:11 in Next.js.
00:00:13 URL stands for Uniform Resource Locator, and its parameters typically consist of several components.
00:00:21 First, we have the scheme.
00:00:22 This is a protocol like HTTP or HTTPS.
00:00:27 Domain is the domain name or IP address of the server, like jsmastery.pro.
00:00:33 Port is optional, like localhost 3000. Then we have a path pointing to a specific resource, like forward slash next15.
00:00:42 We have the query parameters, also known as search params in Next.js, which allow you to pass over additional data to the server as part of the request,
00:00:53 typically used for filtering and modifying the requested resource.
00:00:57 They are appended after the URL with a question mark and separated by ampersands.
00:01:03 For example, question mark parameter 1 is equal to value 1 and parameter 2 is equal to value 2. Finally, you can have a hash indicating some ID on the page.
00:01:15 Now, how can you retrieve this URL information in different ways in Next.js?
00:01:20 Well, if you're in the main page, then you can access the information through the page props, simply saying function page,
00:01:27 where you have params and search params.
00:01:29 So if the URL looks like this, books1234?page2 filter equal to latest.
00:01:37 You can get the ID from params since the params will hold that ID value and search params will hold the value of page and filter.
00:01:46 And that is the key point.
00:01:48 Right here, what we're doing is basically managing state.
00:01:53 In this case, it is local state.
00:01:55 But if you navigate to a different page with these things in the URL, what's going to happen?
00:02:00 You're going to traverse that data over to the second page.
00:02:03 So this can be both local and global state management.
00:02:06 Finally, you can choose to pass those values to other components or use other options to access them.
00:02:13 The best way is to do it through Next.js' hooks, such as useParams and useSearchParams, which give you the same thing, allow you to extract those values.
00:02:22 For example, you can get access to the type if a URL contains the type.
00:02:27 But with this approach, using hooks, it would mean that we're turning that component into a client component.
00:02:33 so you only want to do this for forms or other components that are already client by default.
00:02:38 So as a rule of thumb, if your component is near its parent page, then instead of opting for these hooks, you can pass params and search params of the
00:02:48 page through props to its children components.
00:02:50 A bit of prop drilling won't hurt.
00:02:53 But if your component is far away from the page, then you should use the hooks that I mentioned.
00:02:58 And now you know how to access information from the URL.
00:03:01 But now, how are we actually going to update that information so that it is even there for us to fetch it?