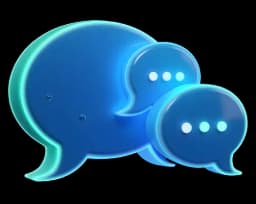
No Comments Yet
Be the first to share your thoughts and start the conversation.
Server Actions in Next.js have revolutionized how we handle server-side operations in React applications. However, there's a common misconception among developers that Server Actions might not be secure.
In this lesson, we'll debunk these myths, explain Server Actions' built-in security features, and provide best practices for maintaining a high level of security.
Misconception
Developers often worry that because Server Actions can be invoked from the client side, they might be susceptible to Cross-Site Request Forgery (CSRF) attacks.
Reality
Server Actions in Next.js have robust built-in protection against CSRF attacks.
How it's actually secure
How to ensure security
Rely on the built-in CSRF protection provided by Next.js.
For large applications with complex architectures, use the serverActions.allowedOrigins configuration in next.config.js to specify a list of trusted origins.
// next.config.js
module.exports = {
experimental: {
serverActions: {
allowedOrigins: ["my-trusted-domain.com", "*.my-trusted-domain.com"],
},
},
};
Resources
Read more about it here
Misconception
There's a concern that error messages or stack traces from Server Actions might expose sensitive server-side information to the client.
Reality
Next.js carefully handles errors to prevent leaking sensitive information.
How it's actually secure
How to ensure security
Always run your application in production mode when deployed.
Implement proper error handling in your Server Actions:
"use server";
import { logger } from "./logger";
export async function sensitiveOperation() {
try {
// Perform sensitive operation
} catch (error) {
// Log the full error server-side
logger.error("Sensitive operation failed", { error });
// Return a generic error message to the client
throw new Error("An error occurred. Please try again later.");
}
}
Resources
Read more about it here
Misconception
Some developers believe that since Server Actions are available on the client, they can be easily manipulated or abused.
Reality
Next.js implements several security measures to prevent tampering with Server Actions.
How it's actually secure
How to ensure security
Keep your Next.js version up to date to benefit from the latest security enhancements.
Implement proper authentication and authorization checks in your Server Actions
'use server'
import { getServerSession } from 'next-auth/next'
import { authOptions } from './auth'
export async function updateUserProfile(userId: string, data: UserProfileData) {
const session = await getServerSession(authOptions)
if (!session || session.user.id !== userId) {
throw new Error('Unauthorized')
}
// Proceed with profile update
// ...
}
Resources
Read more about it here
Misconception
There's a misconception that Server Actions automatically trust all input data, making them vulnerable to injection attacks.
Reality
While Server Actions don't automatically validate all inputs, they provide a clear context for implementing robust input validation.
How to ensure security
Always validate and sanitize all inputs to your Server Actions.
Use a validation library like Zod or Yup for type-safe validation
'use server'
import { z } from 'zod'
const userSchema = z.object({
name: z.string().min(2).max(50),
email: z.string().email(),
age: z.number().int().positive().optional(),
})
export async function createUser(formData: FormData) {
const rawData = Object.fromEntries(formData)
try {
const validatedData = userSchema.parse(rawData)
// Proceed with user creation using validatedData
} catch (error) {
if (error instanceof z.ZodError) {
// Handle validation errors
throw new Error('Invalid input data')
}
throw error
}
}
Implement additional security measures like rate limiting to prevent abuse
'use server'
import { rateLimit } from './rate-limiter'
const limiter = rateLimit({
interval: 60 * 1000, // 1 minute
uniqueTokenPerInterval: 500, // Max 500 users per second
})
export async function createUser(formData: FormData) {
try {
await limiter.check(10, 'CREATE_USER_ACTION') // 10 requests per minute
// Proceed with user creation
} catch {
throw new Error('Rate limit exceeded')
}
}
These are some of the most common myths and misconceptions among developers, but now you’re equipped to understand the reality and take action. Remember, security is a continuous process—stay updated on best practices and regularly review your code to keep it secure as your application grows.
Be the first to share your thoughts and start the conversation.