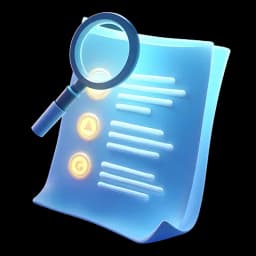
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
Complete source code available till this point of lesson is available at
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:02 To test edit and get question in the client side, we have to actually call it within a form in a similar way we call the create question.
00:00:09 So let's create a new page within which we can use the edit question server action.
00:00:15 We can do that by creating a new route within app, root, questions, ID.
00:00:23 And then right here, we'll create a new folder called edit.
00:00:28 And then within it, create a new page.tsx.
00:00:31 So you'll be able to edit each one of these questions.
00:00:34 And to start this page off, let's actually copy what we have in our create question page.
00:00:39 I believe that is ask question right here.
00:00:42 So let's copy it and paste it right here.
00:00:45 Instead of ask question, we'll rename this one to edit question.
00:00:49 And since we have to know which question we're editing, we can get access to the ID through params by saying params is of a type route params.
00:00:59 Before getting access to the user session, we have to know whether this question exists.
00:01:03 So we can say const destructure the ID is equal to await params.
00:01:10 And then we can say, if an ID doesn't exist, return not found.
00:01:17 This is coming from Next.js navigation, which automatically navigate to a 404 route.
00:01:22 Then we can get access to a session and redirect to sign in if a session doesn't exist.
00:01:27 And finally, we can try to fetch this question's data by saying const data, which we can rename to question as well as the success status of that call
00:01:39 is equal to await get question coming from question actions to which we have to pass an object of question ID equal to id.
00:01:50 Finally, if not success, then we can again return not found.
00:01:55 And we have to do another check.
00:01:56 And that is if question, question mark dot author dot to string is not equal to session, question mark dot user, question mark dot id,
00:02:09 In that case, we need to redirect to just that question details page.
00:02:15 Questions and then ID.
00:02:16 And I think we already have a route created for this.
00:02:19 So that's going to be routes.question.
00:02:23 And then we can pass the ID to that question, which is just equal to ID.
00:02:28 This is to ensure that the currently logged in user is the author of the post.
00:02:32 With that in mind, if all of it is good, We don't have to render an H1 here, ask a question, because this is no longer ask a question form.
00:02:41 Rather, it'll just be a question form on its own, wrapped within a main tag.
00:02:47 To this question form, we'll pass two new things that we haven't passed when we used it as a create form, but now it'll become an edit form too.
00:02:57 So first things first, we have to pass all of the information about the current question that we have, the current question information.
00:03:05 And then we have to pass a new boolean prop called isEdit.
00:03:10 And based on this, we can change some text, like from ask a question to edit a question.
00:03:16 So with that said, let's head over into the question form and let's accept those new props.
00:03:22 That'll be a question as well as isEdit.
00:03:26 By default, we can make it false, but if we do have it, it'll be true.
00:03:30 And this will be of a type params, which we can define right here at the top by saying interface params will contain a question,
00:03:41 optional of a type question.
00:03:45 as well as isEdit optional of a type boolean.
00:03:50 Now let's head over to the question and let's make sure that we have access to content as well.
00:03:56 Because before we just had a title, but now maybe we'll also want to pass some content, which is the body of the question.
00:04:03 So let's make sure that we have it as well.
00:04:05 And finally, we can now modify our form, the question form from being just the create form to also being the edit form.
00:04:13 I mean, just imagine having to redo this entire form for the second time only to function as the edit form.
00:04:19 Imagine all the duplication.
00:04:21 Well, that won't happen here.
00:04:22 I'll teach you how to make this component reusable so you can use it for both create and edit.
00:04:28 First things first, Zod and React hook form make it super easy because we can define the default values.
00:04:36 So right here, I can say that the default value for the title will either be an empty string or question question mark dot title.
00:04:45 So it'll be equal to this if it's already coming from the question we're fetching.
00:04:50 We can do the same thing for the content and we can do a similar thing for the tags by saying something like question Question mark dot tags dot map,
00:05:01 where we get each individual tag by its name or just an empty array of tags.
00:05:06 And finally, where we have the submission form right here, handle create question.
00:05:11 Within here, we can also do the logic for edit.
00:05:14 We'll do it right here above the create question.
00:05:18 And we'll start like this.
00:05:20 If isEdit is true, and if question data exists, in that case, we want to get access to a new result, which will be the outcome of await edit question.
00:05:34 to which we pass the question ID that we want to update equal to question dot underscore ID.
00:05:40 We can add a question mark here in case it doesn't exist.
00:05:43 And then we want to spread out all the data belonging to that question, such as the title, content, and tags.
00:05:50 Now we'll do a very similar thing that we have done right here, and that is show a toast.
00:05:56 So I'll copy this entire if and else part, like before, and move it right here in the edit form.
00:06:03 Within the if, if our result is a success, I'll say something like question updated successfully, and we'll push to the same route as before,
00:06:11 or we'll say something went wrong.
00:06:14 Finally, this is a super important thing, so please don't miss it.
00:06:19 Right before this if ends, you want to have an empty return statement.
00:06:23 Why did we add this return?
00:06:25 Well, that's because if we enter this if right here, that means that we are editing.
00:06:31 And instead of us having to wrap this entire thing in another else or something, you can just return prematurely here, which means that the code,
00:06:39 the execution of this handle create question will actually finish.
00:06:43 And that way this part for create question won't execute.
00:06:47 So that is a very important part to add this return.
00:06:50 If we're doing the edit, we finish the edit and then right there.
00:06:53 And if we're doing the create, we can go until the end.
00:06:56 Perfect.
00:06:57 And then finally, we can head in the button where we're submitting it.
00:07:01 That is here, and we can just change one thing.
00:07:04 Here, instead of just saying ask a question, we can check if isEdit is true, then we can say something like edit, else we can say something like ask a question.
00:07:16 Perfect.
00:07:17 And this is how you make your form reusable.
00:07:19 Now let's go ahead and test all three server actions one more time by first creating a new question.
00:07:25 I'll create a new question with a title of something like, how does the Node.js event loop work?
00:07:31 I'll add a detailed explanation of my problem and I'll add some tags like Node.js as well as JavaScript.
00:07:40 Perfect.
00:07:41 And I'll click ask a question.
00:07:44 This works and we have been successfully redirected to the question details, which we have to implement very, very soon.
00:07:50 But what you can do for the time being is just go to your URL bar and add the forward slash edit right there.
00:07:57 Later on, we'll have a button that points you there, but for now we'll have to do some manual work.
00:08:02 And as you can see, this points you to the same create form, but this time, all of the fields are already pre-populated.
00:08:10 And that allows you to make some quick changes, like maybe adding a few more question marks to make sure that you really get your answers and to show people
00:08:17 that you're super confused.
00:08:18 Or maybe you want to bold some things out to make sure that it stands out.
00:08:22 Whatever it is, you want to apply your changes.
00:08:25 Let's do something very noticeable now so we can truly see the changes we made in our database, such as adding three question marks everywhere.
00:08:34 And maybe we can also change some tags.
00:08:36 Like I will remove JavaScript here and I will add something like, let's do promises.
00:08:42 Perfect.
00:08:43 So now we have two tags, one of which was already there before and the new one.
00:08:47 And let's click edit.
00:08:50 We once again got a new alert saying question updated successfully, and we got redirected to the question details page.
00:08:58 Now, if we had already implemented the question details page, we would be done.
00:09:02 You would already be able to see that everything worked.
00:09:04 But as we haven't built it yet, we can check out the data changes directly in the database.
00:09:10 So either head out to your MongoDB Atlas and then collections, or let me check it out right here by going to questions, documents,
00:09:18 and this is going to be one of those two questions.
00:09:21 I think we're referring to this one.
00:09:23 How does the Node.js event loop work?
00:09:25 We have three question marks here as well as three question marks in all other places, which means that we don't want to wait anymore.
00:09:33 And that basically means that our edit was successful.
00:09:37 Great work.
00:09:39 So to finalize, let's just say implement, edit, and get server actions and implementation.
00:09:49 Let's commit it, sync it, and great job.