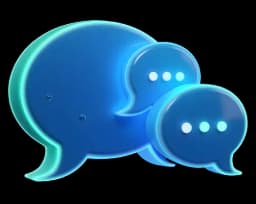
No Comments Yet
Be the first to share your thoughts and start the conversation.
Next.js uses a file-based routing system, making it easy to create routes by creating folders and files. To add a new route like "/about," simply create a folder named "about" with a page.tsx file inside. Nested routes can be achieved by creating folders within folders. Dynamic routes allow for displaying user profiles without creating folders manually. By implementing dynamic routing, users can access specific user profiles within the dashboard. This intuitive system simplifies route creation and organization in Next.js, enhancing the development process for building complex applications.
Be the first to share your thoughts and start the conversation.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we explore the routing system in Next.js, focusing on file-based routing, nested routes, dynamic routes, and layout handling. The tutorial provides step-by-step instructions on creating various routes and reusing layouts effectively.
page.tsx
file in the app directory.error.tsx
files to manage displayed errors.loading.tsx
file to show user-friendly loading indicators during data fetching.00:00:00 Now it's time to dive into routing.
00:00:02 Next.js uses a file-based routing system.
00:00:05 And doing everything on a single page is boring, right?
00:00:08 Currently, we only have one route, which is the home page.
00:00:12 So how do we create a new route, like forward slash about?
00:00:17 It is as simple as creating a new folder and a file.
00:00:21 In the app directory, create a new folder and name it about.
00:00:26 And within that folder, create a new page.tsx file.
00:00:30 Within there, you can create a single functional component that returns a div that says about.
00:00:36 Now go back to the browser and simply add forward slash about to your URL.
00:00:44 And if I zoom it in a lot, there we go.
00:00:47 The route is being displayed.
00:00:49 This is how you create routes in Next.js.
00:00:52 It uses an intuitive file-based routing system, where folders are used to define routes.
00:00:58 The folder name becomes the route name, and the page.tsx inside of it becomes a file that gets rendered for that route.
00:01:05 It's a special file naming convention for displaying that route's content.
00:01:10 But in most cases, that won't be enough.
00:01:13 you'll need nested routes.
00:01:15 Let's say you're developing an admin dashboard within this application and you want to create a route for dashboard users and dashboard analytics.
00:01:24 Of course, you can't create two folders with the same name.
00:01:27 This is where nested routes come in handy.
00:01:30 Let's create a new folder called dashboard, inside of which we'll create two additional folders.
00:01:37 One will be called Users, and another one will be called Analytics.
00:01:42 And both of them will contain a special page.tsx file.
00:01:47 There we go.
00:01:48 You get one, and you get one too.
00:01:51 Within them, we can simply run RAFCE.
00:01:54 If you don't have that quick command, you can install it as a package.
00:01:57 It's called React Snippets.
00:01:59 And then within this one, we can call it Users.
00:02:03 we can duplicate that and we can also add it within analytics and if you go back to your browser go to forward slash dashboard forward slash users you
00:02:13 can see users and if you go to forward slash analytics you can see analytics but of course if you go just to dashboard itself It's going to be a 404. Here's
00:02:24 a quick question for you.
00:02:25 Do you know how you would create a separate dashboard homepage if you wanted to?
00:02:31 The answer is simply add another page.tsx within the dashboard folder itself.
00:02:36 Yes, you can have a dashboard page and then dashboard nested routes.
00:02:41 But this still may not be enough.
00:02:43 In many cases, you'll also need dynamic routes.
00:02:47 What if you want to display each user's profile in the dashboard?
00:02:52 Let's say we have four predefined users in the dashboard users page.
00:02:56 That's going to look something like this, users within a div, and then we have an H1 and a UL with four different users.
00:03:03 Now, let's say you want to show full user details on the page when you click on each one of these users.
00:03:09 Say we want to direct them to dashboard, users, user one, and then dashboard users, user two, and so on.
00:03:18 We can't create these folders manually as we don't know how many users will there be.
00:03:23 This is what dynamic routing is all about.
00:03:26 A route where part of the URL can change or be dynamic, typically based on the user input or specific data in the database.
00:03:34 Creating dynamic routes in Next.js is simple.
00:03:38 All you have to do is wrap the part that changes that is dynamic in square brackets.
00:03:44 In our case, it'll be the user ID.
00:03:46 So let's create a new folder called ID within the users folder.
00:03:52 and make sure to wrap it within square brackets.
00:03:55 That's the rule.
00:03:56 Inside of that file, create a new page.tsx and run RAFCE to create a generic user details page.
00:04:08 Now let's go back to the users page and let's make these users clickable.
00:04:12 Doing that is pretty simple.
00:04:15 We just have to use Next.js' link component.
00:04:19 It looks like this.
00:04:21 You import it from next forward slash link.
00:04:24 You give it an href to where you want to go.
00:04:27 And in this case, let's make it point to dashboard forward slash users forward slash one.
00:04:34 And let's say user one.
00:04:36 We can of course, duplicate this a few more times.
00:04:40 So let's put it within an li and properly close it.
00:04:45 And 1, 2, 3, there we go.
00:04:49 And we can also change their names, 2, 3, 4. Now, if I go back to the browser, I can actually click on these links, and look,
00:04:57 they led me to the user details page.
00:05:00 But they all do, right?
00:05:02 3, 4, they're all leading to the same user details page.
00:05:07 or the page is technically different, because right here, you can see it says Users1, and if you go to Users2, it's going to say Users2.
00:05:16 But how do we extract the different information about each one of the users?
00:05:21 Let me show you.
00:05:22 I'll go back to User Details page, and we can extract this dynamic parameter from the folder, from the params object.
00:05:31 You destructure right here at the top of the function, and then you say params.
00:05:36 Since we're using TypeScript, we also need to define the type of the params.
00:05:39 It'll be an object containing a single ID, which will be of a type string.
00:05:44 And how do I know that it will contain a single property called ID of a type string?
00:05:49 Well, that's because we call this dynamic route ID by saying ID within square brackets.
00:05:56 If you set something like name here, you would be able to extract it by saying something like name.
00:06:01 In this case, let's destructure the ID from params by saying const ID is equal to params.
00:06:09 Or you can also use the dot notation saying params.id.
00:06:13 Finally, now we can say user profile, and then we can show the actual ID.
00:06:20 Let's actually turn this into H1 and give it a class name of text-3xl so we can see it better.
00:06:27 Now, if we go back, you can see user profile 2, user profile 3, 4, and so on.
00:06:34 And in real life, this would work exactly the same.
00:06:38 you will always be fetching just the user ID.
00:06:40 But then, from that page, you can make a call to the database that'll give you all the other user information, such as their avatar,
00:06:48 full name, username, and more.
00:06:50 So, now you know what params are.
00:06:53 And if you want to get access to this information, such as this ID, elsewhere and not within a Next.js page, you can use a client component hook called
00:07:03 UseParams that lets you read routes dynamic parameters filled in by the current URL.
00:07:08 Next, let's learn about layout.
00:07:10 Earlier, I told you that the layout.tsx file is the main starting point of our app.
00:07:16 To demonstrate this, let me add a text of Root before rendering the children.
00:07:21 I'll do that right here.
00:07:23 And once again, I'll make it H1 with a class name of text-3xl and I'll say Root.
00:07:32 Now, if you check out the homepage, it'll say Root.
00:07:35 Welcome to Next.js.
00:07:37 Then if you go to forward slash about, you'll see Root about.
00:07:41 or if you go to dashboard forward slash users, if it doesn't appear automatically for you, you might need to reload your server by opening up the terminal,
00:07:50 pressing Ctrl C to stop it, and then rerunning npm run dev.
00:07:55 But you get the point.
00:07:56 Root appears on top of all the pages.
00:08:00 This is because layout.tsx acts as the parent for all of these routes.
00:08:07 It allows you to share UI elements across multiple pages.
00:08:11 For example, you can place features like a navbar or a footer in the layout, and they'll appear on all children routes, saving you from having to import
00:08:21 them on every page.
00:08:23 Simply add them once in layout.tsx, and they'll be available wherever needed.
00:08:29 In Next.js, a root layout is always required, but you can create additional layouts if necessary.
00:08:36 Let's say you want to render a specific UI just for the dashboard routes.
00:08:41 We can do that by adding another layout.tsx right here within the dashboard.
00:08:48 The name has to be layout.
00:08:50 Don't try something else, otherwise Next.js won't recognize it.
00:08:54 Creating a new layout is as simple as creating a new functional component and then getting children right here.
00:09:01 Just to make TypeScript happy, you can also define additional types such as children is equal to React node, and you might need to import React.
00:09:10 There we go.
00:09:11 Now everybody's happy.
00:09:14 Within this div, we can then render children, and the children in this case will be all other pages that you're showing within this layout.
00:09:22 But if you want to add some kind of a special dashboard navbar, so let's say dashboard, then you can add it to the layout.
00:09:29 And let's also apply the same styles.
00:09:32 by giving it a class name equal to text-3xl.
00:09:35 So now if you reload, you can see root.
00:09:39 This is coming from the root layout, and then dashboard is coming from the dashboard layout.
00:09:43 Just imagine that these are different pieces of the UI that we're showing on different screens.
00:09:48 So dashboard users and dashboard analytics has both root and dashboard, but as soon as you exit the dashboard route group,
00:09:57 you're back to just root.
00:09:58 I hope this makes sense.
00:10:00 But there's more.
00:10:02 Route groups.
00:10:04 Let's say you don't want the route layout to appear in the dashboard routes and only show up in non-dashboard routes like home,
00:10:12 about, and more.
00:10:13 This means that you'd need a separate navbar for the dashboard and another one for the non-dashboard routes.
00:10:19 That's pretty similar how things work in real life, right?
00:10:23 In such scenarios, you can use route groups.
00:10:26 They allow you to organize your route segments and project structure without impacting the URL path.
00:10:33 This means that you can create folders, but unlike nested routes, they won't show up in the URL.
00:10:39 You do that by wrapping the folder name inside parentheses.
00:10:43 In our case, let's create two different folders.
00:10:46 One will be called dashboard in parentheses and the other one will be called root in parentheses as well.
00:10:54 Now, move the dashboard folder within the dashboard in parentheses and move the homepage and the about folder in the root.
00:11:04 Now, remove the root from the root layout file.
00:11:08 This, let's say this is a root navigation bar.
00:11:11 And add another layout.tsx file within the root route group.
00:11:16 Once again, it is a single functional component that accepts children.
00:11:21 And then now you can render the navbar right here.
00:11:24 So let's do an H1.
00:11:26 In this case, let's give it a class name equal to text-3xl.
00:11:31 And let's simply say navbar.
00:11:33 And you must render children in every layout file.
00:11:37 So let's render them below.
00:11:38 And one last change is to move the layout.tsx from this dashboard folder to this dashboard folder right here.
00:11:48 Now, each route group has its own layout.
00:11:52 Now, if you go back to the website, you'll see everything is as it was.
00:11:57 We have navbar on top of the home and forward slash about route.
00:12:01 And if we go to dashboard and then users, you can see that we have only a dashboard navigation bar.
00:12:08 Everything works as expected.
00:12:10 So why are we using these route groups in the first place?
00:12:13 Well, take a look.
00:12:15 They're making our code more organized without affecting the URL.
00:12:20 To go to the home page or to the about page, you don't have to say forward slash root, forward slash about.
00:12:27 You simply say about.
00:12:28 So whenever you place a folder name within parentheses, that means you're creating a route group and it will not be mapped to a URL path.
00:12:37 And why is this useful?
00:12:38 Well, one, for organizing your code.
00:12:41 You can see everything is cleaner now.
00:12:43 And the second reason is that you can have separate layouts for different route groups, which are going to give you a different UI,
00:12:50 but without affecting the URL.
00:12:52 So let's rewind one more time.
00:12:55 Route groups allow you to create folders without affecting the URL.
00:13:00 And even though creating route groups isn't taken into account, you can still create files like layout.tsx inside of them.
00:13:07 And it's important that this root route group still points to the homepage.
00:13:12 So this page right here is the primary homepage.
00:13:15 If you were to copy and paste this file into the dashboard, That wouldn't work, because now we have two parallel routes that resolve to the same path.
00:13:24 I hope this is clear.
00:13:26 Understanding route groups is extremely useful, because they allow you to separate concerns, manage route segments, and render content properly,
00:13:36 all without showing them in the URL.
00:13:38 Next, let's talk about error handling.
00:13:41 In Next.js, there's a special file called Error.js, or TS if you're using TypeScript.
00:13:48 that catches errors and displays them on the UI.
00:13:52 Similar to how we were able to create multiple layout files for each folder, be that a route group or a route folder, we can do the same for the error.ts file.
00:14:01 Let's throw an error on purpose in the About page.
00:14:04 I'll head over to that page and I'll say throw new error.
00:14:11 I'll say something like not implemented.
00:14:14 And don't forget to delete this duplicate of a page.tsx within the dashboard.
00:14:18 That one is still throwing us the old error.
00:14:21 Once you do that and go to about, you'll see an error that is fine for us developers, but you never want to show it to the end user.
00:14:30 Rather, if there has to be an error, let's show it within a nice UI.
00:14:33 At least the user will feel better that way.
00:14:36 To do that, let's create a new error.tsx file in the root folder.
00:14:42 Error.tsx In it, I'll copy the code provided to us by the Next.js documentation.
00:14:48 This component is just nicely handling the error.
00:14:52 Save and revisit the About page.
00:14:55 Check it out, we don't see that red popup anymore.
00:14:58 Rather, we see what we have implemented in the error.tsx file.
00:15:02 Something went wrong, try again.
00:15:05 Super simple and straightforward to handle errors in Next.js, right?
00:15:09 And as I said, you can even create these error files specific to different routes.
00:15:14 If you just want to have one global error, then you can do it by creating a globalerror.tsx file in the root of the app folder.
00:15:22 That's going to look something like this.
00:15:24 I'll go into the app and create a new file called global error.tsx.
00:15:34 The code will look something like this.
00:15:35 I'm not sure why my indentation is not working when copying, but you get the idea.
00:15:41 You can specify the HTML and the body and simply show some kind of an error.
00:15:46 Take a note that error boundaries must be client components.
00:15:50 Now, going back to our app, can we see this error?
00:15:54 Well, not really.
00:15:55 Unlike layout.tsx, which displays everything from its parent, the error file works differently.
00:16:02 Only the closest error file to the route takes priority.
00:16:06 Meaning, you won't see the content both from global error.tsx and from the root error.tsx.
00:16:14 Errors will bubble up to the nearest parent error file.
00:16:17 Not all of them.
00:16:19 Makes sense?
00:16:20 And to talk about something else other than errors, let's talk about loading UIs.
00:16:26 Loading UIs in Nix.js works very similarly to error handling.
00:16:31 You want to show some kind of a loading progress while data is being fetched for users with a slow internet connection.
00:16:38 It is as simple as adding a new loading.tsx file in the folder.
00:16:45 In there, you can create any kind of a loader or a nice-looking spinner.
00:16:51 Now, as your page reloads, this file will show.
00:16:54 I'll keep reloading the screen so you can see that loading bar.
00:16:57 Adding loadings to your Next.js really doesn't get simpler than that.
00:17:01 While the data is loading, Next.js will show the content you specified in the loading file.
00:17:07 And once the data is received, the content in the page you're trying to render will be shown.
00:17:12 It's super useful and surprisingly easy to do.
00:17:15 And in addition to these main file conventions like page, layout, error, and loading, Next.js also provides other features like parallel routes,
00:17:24 intercepting routes, and localization routes that display content based on the user's language.
00:17:31 You can explore all of these in the Next.js's documentation.