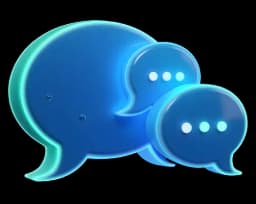
No Comments Yet
Be the first to share your thoughts and start the conversation.
Okay, Now that you're familiar with the entire Next.js application structure, let’s get our hands dirty.
At the start of the crash course, we talked about Architecture, where we can write two types of components: Client And Server Components.
If you remember, I said by default the React components you’ll write are Server Components. But are they?
We have this home page component saying, “Welcome to Next.js.” Let’s add a console log before we return the jsx.
page.tsx;
export default function Home() {
console.log("Which component am I?");
return (
<main>
<h1 className='font-bold text-3xl'>Welcome to Next.js</h1>
</main>
);
}
Where do you think this console log will show up? In the browser?
Nah, interesting, right? It will actually appear in your terminal, not in the browser. Check your terminal—that's where your log is. This is what a Server Component is; it's rendered on the server, not in the browser.
React Server Component (RSC)
Server components are rendered on the server, and their HTML output is sent to the client. Since they’re rendered on the server, they can access server-side resources directly, like databases or the file system. This helps reduce the amount of JavaScript sent to the client, improving performance.
Server components are excellent when:
All right, but if server components are better, why can’t everything be a Server Component?
Well, if your component requires browser interactivity, such as clicking buttons, navigating to different pages, and submitting forms, then you need to turn it into a client component.
So, what are React Client Component (RCC)
Client components are rendered on the client side (in the browser). To use them in Next.js, you must add the "use client" flag at the top of the component.
Let’s create a new component, say hello.client.tsx inside the components folder.
hello.client.tsx;
("use client");
function Hello() {
console.log("I am a Client Component");
return (
<div>
<h1>Hello</h1>
</div>
);
}
export default Hello;
After this, import this into the app/page.tsx file and see where this Client Components log appears.
page.tsx;
import Hello from "@/components/hello.client";
export default function Home() {
console.log("Which component am I?");
return (
<main>
<h1 className='font-bold text-3xl'>Welcome to Next.js</h1>
<Hello />
</main>
);
}
Let’s check the terminal—no, browser—and there is the log. hello.client.tsx is actually a client component.
But wait, you did see something in the terminal, too, right? The log of the client component is also there. How? Or maybe, more importantly, WHY?
This is because Server Components are rendered only on the server side, while Client Components are pre-rendered on the server to create a static shell and then hydrated on the client side.
This means that everything within the Client Component that doesn't require interactivity or isn't dependent on the browser is rendered on the server. The code or parts that rely on the browser or require interactivity are left as placeholders during server-side pre-rendering. When this reaches the client, the browser renders the Client Components and fills in the placeholders left by the server.
I hope that’s crystal clear.
Be the first to share your thoughts and start the conversation.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we explore the differences between server and client components in Next.js. The discussion emphasizes the architecture and rendering process of these components, highlighting when to use each type effectively.
useClientDirective
.00:00:00 Okay, okay.
00:00:01 Now that you're familiar with the entire Next.js application structure, let's get our hands dirty.
00:00:06 At the start of the crash course, I was telling you a bit about architecture, where you can write two different types of components,
00:00:13 client and server components.
00:00:16 If you remember, I said that every component you create, Next.js will by default turn into a server component.
00:00:23 But is that really the case?
00:00:25 Here on the homepage, we have a component saying, welcome to Next.js.
00:00:29 Let's add a consa log before the return statement.
00:00:33 Consa log?
00:00:34 What am I?
00:00:35 I was about to start, what am I?
00:00:37 Server or client component?
00:00:39 But it looks like our AI autocomplete is having a bit of an existential crisis.
00:00:43 It's saying, what am I doing here?
00:00:46 But yeah, I'll just add server or client.
00:00:49 Let's see, which type of component is this?
00:00:52 By default, we have absolutely nothing here.
00:00:55 Just export default function home where we have a console log and an H1 statement.
00:01:00 Where do you think this console log will appear?
00:01:02 Do you think we'll be able to see this console log in the browser?
00:01:04 Well, up to this point, I would tell you no, it's not gonna appear in your browser because this is a server-side component,
00:01:11 but would you look at this?
00:01:13 Next.js team knew that some of us might be searching for this console log in the browser, so even though technically it shouldn't be showing here,
00:01:20 they're still showing it to us, but they have added this server tag at the top left, so we know it's actually rendering from the server.
00:01:28 Pretty cool, Next.js.
00:01:29 But if you actually knew what you were doing, you would immediately go to the terminal to find your console log.
00:01:35 And here it is.
00:01:37 So now we know that this is actually rendering on the server.
00:01:41 Server components are rendered on the server.
00:01:43 So this brings me to the topic of React server components.
00:01:47 They're the components that are rendered on the server, and their HTML output is then sent to the client.
00:01:53 Since they're rendered in the server, they can access server-side resources directly, like databases or the file system.
00:02:00 This helps reduce the amount of JavaScript sent to the client, improving performance.
00:02:06 Server components are excellent when you need direct access to server-side resources, like accessing files in a file system,
00:02:14 or you want to keep sensitive information, well, sensitive, such as access tokens and other keys, safe on the server.
00:02:21 Alright, but if server components really are better, why can't everything be a server component?
00:02:28 Well, if your component requires browser interactivity, such as clicking buttons, navigating to different pages, and submitting forms,
00:02:36 then you need to turn it into a client component.
00:02:39 So, what are React client components?
00:02:42 Client components are, of course, rendered on the client side.
00:02:46 And in this case, ClientSite simply means the browser.
00:02:50 To use them in Next.js, you must add a useClientDirective at the top of the component.
00:02:56 In our case, let's do that by creating a new folder within the app folder and call it components.
00:03:03 And within it, create a new file called hello.tsx.
00:03:08 Right at the top, you can add that useClientDirective that I was telling you about.
00:03:13 And then you can simply create a new functional component called hello that simply returns a div with an h1 that says hello.
00:03:21 And I've also added a console log saying, I am a client component.
00:03:27 See, this one doesn't have an existential crisis.
00:03:29 It knows what it is.
00:03:30 Now that you've created it, let's go back to the page.tsx.
00:03:34 Let's wrap this h1 within a react fragment.
00:03:38 allowing us to add another adjacent element.
00:03:41 In this case, we'll simply import the hello component, coming from add forward slash app forward slash components forward slash hello.
00:03:49 Now, let's check the terminal.
00:03:51 Okay, we don't see that console log there, but that makes sense, right?
00:03:56 Because hello knows it's a client component.
00:03:59 But if we go into the browser, there we go.
00:04:01 I am a client component coming from hello.tsx.
00:04:06 But wait, I think I initially missed it, but now I see it.
00:04:09 I Am A Client component is so confident that it appears right here too, but why, or how is that happening?
00:04:16 Well, this is because server components are rendered only on the server side, while client components are pre-rendered on the server side to create a static
00:04:28 shell and then hydrate it on the client side.
00:04:30 This means that everything within the client component that doesn't require interactivity or isn't dependent on the browser is still rendered on the server.
00:04:40 The code or parts that rely on the browser or require interactivity are left as placeholders during server-side prerendering.
00:04:48 When they reach the client, the browser then renders the client components and fills in the placeholders left by the server.
00:04:56 I hope that makes sense.
00:04:57 And that answers the question of what is server-side prerendering.
00:05:02 A pretty cool feature by Next.js.
00:05:04 I hope that's clear now.
00:05:05 But if you're still unsure, take a second to pause it and re-watch it until it clicks.
00:05:11 You can also add some additional cons logs or components and then render them within the page.tsx.
00:05:17 Don't proceed further if that doesn't make sense.
00:05:19 And for a deeper dive with clearer explanations and more visuals, I would recommend checking out the Ultimate Next.js course.
00:05:27 where I break down the entire client-server architecture in detail.
00:05:32 So, finally, when should you allow Next.js to turn your components into server-side components, and when should you manually change them to client-side?
00:05:40 Well, a good rule of thumb is to leave it as server-side component until you need some browser interactivity, at which point you'll most likely get an error,
00:05:48 and then you can add the use-client directive at the top.
00:05:51 But if you want to understand on a deeper level when should you use each one, always refer to this table within the Next.js docs.
00:05:59 I'll make sure to link it as an essential resource within the ebook that you can download.
00:06:03 This is the best table that simply explains when you need something to be a server or a client component, depending on what you need that component to do.