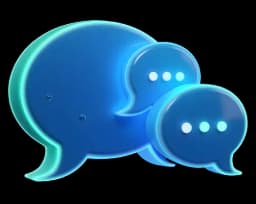
No Comments Yet
Be the first to share your thoughts and start the conversation.
The video discusses data fetching in Next.js, comparing client-side and server-side fetching methods. Client-side fetching involves more code and slower initial load times, while server-side fetching offers cleaner code, faster responses, and improved SEO. Server-side fetching reduces time to first contentful paint and allows search engines to index content more easily. Additionally, server components simplify data fetching logic by keeping it closer to the data source. Overall, server-side fetching in Next.js provides a better developer experience, faster load times, and improved SEO benefits compared to client-side fetching.
Be the first to share your thoughts and start the conversation.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
In this lesson, we explore the various methods of data fetching in Next.js, highlighting the benefits of server-side fetching over client-side fetching. The discussion emphasizes the importance of optimizing performance, improving SEO, and enhancing developer experience through efficient data management strategies in Next.js applications.
useEffect
to make asynchronous fetch requests, leading to a less efficient loading process.00:00:00 data fetching, the most interesting concept in Next.js.
00:00:04 There are different ways in which we can fetch content.
00:00:08 A traditional way is using a use effect.
00:00:11 For example, this is what you would do.
00:00:14 Let's say that you have a homepage where you're trying to set and fetch some albums.
00:00:19 You create a new empty use state.
00:00:22 You declare a use effect within which you declare an asynchronous fetching function.
00:00:29 Within it, you have an await fetch where you're trying to get some albums, and then you return that JSON and set it to this state.
00:00:37 Finally, going a bit down, you map over those albums and show the data.
00:00:42 But this isn't super efficient.
00:00:44 There is a better alternative to fetch, and it's happening on the server side.
00:00:49 Not only is it faster and more efficient, the code is also so much cleaner.
00:00:54 Check this out.
00:00:55 You declare a new functional component called home and immediately on top of it, you make a fetch request.
00:01:02 You check if it fails.
00:01:03 If it doesn't, you simply declare it and set its response.json to the albums variable.
00:01:10 You map over it and display the details.
00:01:13 Go ahead, pause this video and test it out.
00:01:16 You don't necessarily have to do all the styles, but you can at least try to fetch the data, map over the albums, and show each album's title.
00:01:24 If you do it, visit the website and see if it works.
00:01:28 You'll not see anything.
00:01:30 You'll have to refresh to see the result.
00:01:32 And it's not because HMR is not working.
00:01:35 but rather, HMR is caching your content.
00:01:39 In latest versions of Next.js, there is a new feature called Server Components HMR Cache that allows you to cache fetch responses in server components
00:01:51 across hot module replacement refreshes in local development.
00:01:54 What this means is you'll have faster responses and reduced costs for build API calls.
00:02:01 But now, coming back to the difference between server-side fetching versus client-side fetching, first of all, you'll notice the code difference between
00:02:09 the two strategies.
00:02:10 With server-side fetching, you've wrote fewer lines of code, which improves the DX, Developer Experience.
00:02:18 But that's not the only benefit.
00:02:20 Server-side fetching has so many more benefits, like improved initial load time.
00:02:25 As a server-side data fetching allows the page to be rendered with the data already included, it reduces the time to first contentful paint,
00:02:34 FCP.
00:02:35 In the client-side example, the user sees an empty page until the data is fetched and then rendered.
00:02:41 The second and most important benefit of server-side fetching is better SEO.
00:02:48 Search engine crawlers can more easily index content rendered on the server as the content is already provided in HTML format.
00:02:57 In contrast, client-side fetching may result in content not being visible to crawlers right away, since it first needs to be fetched and then displayed
00:03:07 on the UI, which can negatively impact your SEO.
00:03:10 Next, not only is the code shorter, but the logic is simplified.
00:03:17 Server components allow you to keep data fetching logic on the server closer to your data source.
00:03:23 This can simplify your component logic and reduce the need for use effect and use state hooks.
00:03:28 And the next benefit is not that easy to understand that first, and it is automatic request deduplication.
00:03:36 Next.js provides that automatic request deduplication when fetching data on the server, which can improve performance and reduce unnecessary API calls.
00:03:46 Basically, request deduplication makes sure that when the same data is requested multiple times at once, only one request is sent.
00:03:55 It stops duplicate requests from being made.
00:03:58 And when talking about benefits of the server-side fetching versus client-side fetching, I really can't miss on improved security.
00:04:07 Keeping API calls on the server allows you to better protect sensitive information like API keys, which should never be exposed on the client side.
00:04:17 And adding to the list of benefits, we have reduced network waterfall.
00:04:21 Client-side data fetching often leads to a network waterfall where requests are made sequentially.
00:04:27 Server-side fetching can more efficiently parallelize those requests.
00:04:32 I'll teach you how to do these parallel requests in our build and deploy project, so keep watching.
00:04:38 But this isn't just about fetching.
00:04:40 You can server render any other calls.
00:04:43 Maybe a direct database call to Prisma to get the list of posts.
00:04:47 Or even with Mongoose and MongoDB, like this.
00:04:50 That's the beauty of Next.js.
00:04:53 This is achievable because these components are React server components, which allow you to access server-related resources directly.
00:05:02 This means we can make direct database calls instead of needing to create an API and then fetch that API once again.
00:05:10 And if you're still not convinced, you can always go for client-side fetching.
00:05:15 How?
00:05:16 Just add use client to the top, bring back the use effects, the use states, and you're all set.
00:05:21 Next.js will allow you to do whatever you think is best.
00:05:24 And let's be honest, this is how we all used to use Next.js when we first transitioned over from React.
00:05:31 It only feels natural, but it is the wrong way to use Next.js.
00:05:36 And that's what made me create the whole ultimate Next.js course in the first place.
00:05:40 To teach Next.js the right way.
00:05:43 But now, take a moment to test both client-side and server-side rendering.
00:05:48 You'll notice the differences I mentioned, such as seeing the blank page initially with client-side rendering, or if you inspect its source and see that
00:05:56 nothing is there, or client-side not functioning at all if you disable JavaScript.
00:06:00 In contrast, server-side fetching will still display the results even if JavaScript is turned off in the browser.
00:06:08 But now that we know that server-side fetching is preferable, let's explore different server-side strategies that allow you to manage how and when you
00:06:18 render your content on the server-side.
00:06:20 You see, this is a crucial part of Next.js.
00:06:24 And I know that some of you might be thinking, I just want to code already.
00:06:28 But without understanding these concepts, you'll probably end up saying, I hate Next.js, it never works.
00:06:35 The truth is, it does work.
00:06:38 Just not for you.
00:06:39 You just need the right skills to make it happen.
00:06:41 That's why, even on my platform, I dedicate an entire section to breaking down these strategies before we touch a single line of code.
00:06:50 And we'll be doing the same thing in this video.
00:06:53 Trust me, it'll make your life a whole lot easier.
00:06:56 The first technique is static site generation, or SSG.
00:07:02 a technique where HTML pages are generated at build time.
00:07:07 This means that the content is created when you deploy your site, not when a user requests it.
00:07:14 It's extremely fast and can be served from a CDN, but it's not suitable for websites that need frequently updated content.
00:07:22 By default, Next.js uses a static rendering strategy.
00:07:26 Your result is cached and delivered through a CDN network.
00:07:31 Ideally, you want to use this strategy for blogs, documentation sites, or marketing pages.
00:07:37 But in many cases, you'll have to go for Incremental Static Regeneration, or ISR for short.
00:07:45 It's an extension of SSG that allows you to update static content after you've built your site.
00:07:51 This means that it'll create static pages at build time behaving like SSG, And then after some time has passed, it'll create or update those static pages
00:08:03 once again after you've deployed your site.
00:08:05 Basically, it combines the benefits of static generation with the ability to refresh or update content.
00:08:13 If you want to use ISR as a strategy, you can do this in two ways.
00:08:18 The first one is time-based revalidation.
00:08:21 You can revalidate your entire page after some time by simply exporting a variable called revalidate and set it equal to the number of seconds of when
00:08:31 you want to revalidate.
00:08:32 Isn't that crazy?
00:08:34 Expert cons revalidate and then that changes your rendering strategy.
00:08:38 Super simple.
00:08:39 And the second way is to revalidate the request after some time.
00:08:43 And that would look something like this.
00:08:45 You have a fetch and then you provide the options object with next revalidate.
00:08:51 The difference between these two is that the first approach uses the revalidate export, which is a route segment config.
00:09:00 It sets the revalidation time for the entire page to 3600 seconds, or 1 hour.
00:09:06 This means that the entire page, including all data fetches within it, will be revalidated every hour.
00:09:13 While the second approach uses the next revalidate option in the fetch function.
00:09:18 It sets the revalidation time specifically for this data fetch to 1 hour.
00:09:23 This means that only this particular data fetch will be revalidated every 3600 seconds, while other parts of the page or other data fetches,
00:09:34 if any, are not affected at all.
00:09:37 And there's also on-demand validation.
00:09:39 Instead of doing it on time, you can also revalidate using Revalidate Path and Revalidate Tag for on-demand validation of content.
00:09:50 I hope that's clear enough.
00:09:51 That's how you can use ISR.
00:09:54 It's perfect for those websites whose content changes occasionally, but still doesn't need real-time updates.
00:10:01 The next rendering strategy is Server Side Rendering, or SSR.
00:10:07 It generates the HTML for a page on the server in response to a user's request.
00:10:14 This means that the content is created dynamically for each user request, and not only for each deployment.
00:10:21 It's slower than SSG and puts more load on the server, but you'll always have up-to-date content.
00:10:28 This is ideal for highly dynamic content or pages that need real-time data.
00:10:34 Similar to what we did with ISR, you can use the same configs for SSR.
00:10:38 For time-based revalidation, instead of setting it to some random number of seconds, you simply set it to 0, telling Next.js to render the page every time
00:10:47 the user requests it.
00:10:48 And if you only want to do it for specific requests, You can use the cache no store or set the revalidate to zero there.
00:10:56 And same thing happens with the on-demand validation, like revalidate path and revalidate tag.
00:11:01 You can learn more about them in the documentation.
00:11:04 But so far, everything is super intuitive.
00:11:08 And finally, there's the partial prerendering, PPR.
00:11:12 It's a new rendering model that combines static and dynamic rendering.
00:11:17 It allows you to render a static shell of a page while streaming dynamic content.
00:11:23 The key difference with PPR is that it allows for a hybrid approach within a single page, unlike other strategies that work on a page level.
00:11:33 Here's how PPR works.
00:11:35 During build time, Next.js generates a static shell of the pages.
00:11:40 This shell includes the layout and any static parts of the page in the form of components.
00:11:46 This static shell includes placeholders for dynamic content.
00:11:51 We do it by wrapping dynamic components in a suspense tag.
00:11:55 When a user requests the page, the static shell is served immediately, and then the dynamic content is streamed in as it becomes available.
00:12:05 So what does this mean?
00:12:06 Well, with PPR, you can have static components, dynamic components, and components that are partially static and partially dynamic,
00:12:15 depending on what you need.
00:12:17 you'll actually learn about all of these strategies in action in the application you'll develop today.
00:12:23 So for now, let's keep going.
00:12:24 We're almost there and ready to start building the app.