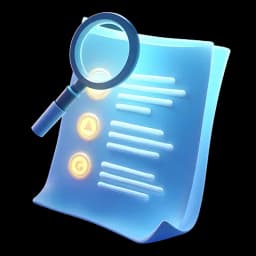
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
The video discusses routing in Next.js compared to React Router DOM. Next.js simplifies routing with a file-based system, where folders define routes and files create UI. The process is demonstrated by creating folders for About and Contact pages, each with a Page file.
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:00 Now, let's dive into routing.
00:00:03 One of Next.js' cool features is its ability to handle routes out of the box.
00:00:08 But before we jump into that, let's first understand how routes are created in React.
00:00:15 Here's an example of how a route can be created using React Router DOM version 6 in React.
00:00:22 We first import all the necessary packages from React Router DOM.
00:00:27 Of course, after installing it as a separate package, then we initialize our app, which is a functional component, wrap everything in a router,
00:00:36 routes, and then we create a new route with a path and element for each one of the pages we want to have within our application.
00:00:44 And here's an example of a nested dynamic routing for a multi-page website like an e-commerce site.
00:00:52 As you can see, things get complicated pretty soon.
00:00:55 And this app isn't even that big.
00:00:58 Scary, isn't it?
00:00:59 Not only do we need to download and handle an external package, but as our application gets bigger, the routing becomes more complicated,
00:01:08 making it harder to manage and understand.
00:01:11 Now, let's explore what Next.js brings to the table when it comes to routing.
00:01:16 Next.js aims to simplify the process by using a file-based routing system.
00:01:22 And what does that mean?
00:01:24 Well, folders are used to define routes and files are used to create UI for that route segment.
00:01:33 For instance, to convert the previous React.js example into Next.js, we only need to create two folders named About and Contact,
00:01:43 and then we'll create a special file associated with that route segment inside each folder, a file called Page, with an extension of js,
00:01:52 jsx, or tsx.
00:01:55 Now, let's understand it while we code.
00:01:58 Quickly create a new folder, and we can call it routing, and then drag and drop it to your empty Visual Studio code.
00:02:06 While in there, go to view and then terminal to open up a built-in integrated terminal.
00:02:12 And we can quickly spin up our Next.js application by running mpx create-next-app add latest and then dot slash and press enter.
00:02:22 It's going to ask us a couple of questions.
00:02:24 For now, let's say yes for Tabscript, no for ESLint, yes for Tailwind, no for Source, yes for Router, no for Alias, and this is it.
00:02:34 I just press enter on all of them.
00:02:37 This is the default installation process.
00:02:40 This is going to install all the necessary packages we need and no additional package for routing.
00:02:45 That's important.
00:02:47 Once the packages get installed, you'll notice a basic file and folder structure.
00:02:52 Now, let's clean it up a bit.
00:02:54 Go to the globals.css file, delete everything that's there, and simply add a couple of properties.
00:03:00 Main, padding to rem, display flex, justify content center, align item center, and flex direction column.
00:03:10 Then you can go within your page.tsx and remove everything, including this main tag.
00:03:18 This is all just basic boilerplate from Next.js.
00:03:22 And the only thing we want to have in there is going to be a main tag that's going to have an h1 of home.
00:03:28 Now let's simply save this file and run it by running npm run dev to ensure that application is working correctly.
00:03:37 And there we go.
00:03:38 You can see home right here.
00:03:41 Now, let's create a simple navigation bar component to move between different pages.
00:03:46 Create a new components folder inside of the root of the application.
00:03:52 and inside create two files.
00:03:55 One is going to be called navbar.jsx and the second one is going to be called navbar.module.css.
00:04:06 Within our navbar folder, I'm going to paste the code that you can also find below this lecture.
00:04:13 It's going to be a simple navbar component.
00:04:16 In case you want to follow along, feel free to pause this video and type it out by hand.
00:04:21 We first have to import some things right here.
00:04:24 But you can also just listen to me and understand the concepts that way.
00:04:28 Or you can copy this and then replicate the entire application and then go through it and change some things around to fully understand what's happening.
00:04:35 But still, I'm going to explain every single little detail.
00:04:39 First, we want to import the link component from NextLink.
00:04:43 First, we want to import the link component from NextLink.
00:04:47 And we also import some styles so that our application looks better while we showcase it.
00:04:52 Then, we create an HTML5 semantic tag called header and nav.
00:04:57 And most importantly, we create an unordered list of links where we have each individual link pointing to a different path in our application.
00:05:06 And below, you'll also be able to find the styles.
00:05:09 We're simply styling a navigation bar here.
00:05:12 Nothing too complex.
00:05:14 But now, to ensure that this navbar is appearing on all route pages, we have two options.
00:05:20 The first option is to import the navbar in each route page.
00:05:25 For now, we only have one, but imagine if our app grows, it's going to be hard to import the navbar into every single page.
00:05:32 And keep in mind, that's how we have been doing it in React so far.
00:05:36 And the second option is to import the navbar component in the parent component of these routes, such as layout.tsx.
00:05:44 Importing it into the layout component will consistently display it across all routes.
00:05:49 So going to the page, importing it right here at the top of the page and then displaying it as a self-closing component right here within the main is the
00:06:00 wrong choice.
00:06:01 We don't want to do that.
00:06:03 because then once we create a new page, we would have to replicate this entire thing.
00:06:07 Not ideal.
00:06:08 So the right method is to go within the layout.tsx, import the navbar right here, and then call it right here within our body.
00:06:19 This is now going to appear in all of our pages.
00:06:22 And by now, if you have followed all the steps properly, you should see this inside your browser.
00:06:27 Next.js, and then these three links on the right side.
00:06:31 And now it is routing time.
00:06:33 To create a route for the about page using the file-based routing system, we need to create an about folder inside of the app directory.
00:06:42 It is important to ensure that the file name inside this folder is lowercase, as the name of this folder will be exactly the same as the route.
00:06:52 So let's create an about section.
00:06:55 After creating the folder with the name about, create the special UI file called page.js or JSX, or in this case we're using TypeScript,
00:07:05 so TSX is fine as well.
00:07:08 And there, we'll simply need to export default function page, we'll return main H1, but this time it's going to say about.
00:07:18 Well, that's it.
00:07:19 Go to your browser and click the About link in the nav bar.
00:07:24 You will notice that the URL changes and the text displayed switch from home to About.
00:07:30 How easy is that?
00:07:32 Especially compared to the ReactJS code we examined at the start.
00:07:36 Now go ahead and create a route for contact in the same way.
00:07:41 Within the app directory, create a new folder called contact.
00:07:45 Within it, create a new page called page.tsx.
00:07:51 And once again, export default function, contact, contact.
00:07:56 After saving the changes and returning to the browser, if you click contact, you get redirected.
00:08:03 and you didn't even have to reload the page.
00:08:06 All modifications are immediately visible without the reload, all thanks to Next.js' fast refresh feature.
00:08:13 That was all about creating a simple route in Next.js.
00:08:17 But what about nested or dynamic routes?
00:08:20 What do we have to do?
00:08:22 Well, let's explore.
00:08:23 Nested routes are as simple as nesting one folder inside of another.
00:08:28 For instance, we wish to set up a route named projects forward slash list.
00:08:33 To achieve this, we can create two folders.
00:08:36 The first one is going to be called projects and within it, another folder called list.
00:08:44 And then inside of the list, we add that page dot TSX file containing the interface for the project list.
00:08:55 And to simplify the navigation to this page, let's quickly include a link tag within the home page.
00:09:02 So inside of our home page, right here, we can add a new link tag, which we can import from next link.
00:09:12 It's going to say C projects and we can give it an href equal to forward slash projects forward slash list.
00:09:22 If we save this and now visit the homepage, you'll see C projects and clicking on this link will take you to the route we created,
00:09:32 which is forward slash projects forward slash list.
00:09:36 And here you can see it in the URL as well.
00:09:39 So, simply nest folders within one another and create whatever route you want.
00:09:45 Moving forward, we have dynamic routes.
00:09:48 Think of it as nested routes, but with a slight difference.
00:09:52 Unlike traditional nested routes, where we need to know the exact route name in advance, dynamic routes allow for more flexibility.
00:10:01 The route is determined based on changing data in the application, so we don't need to predict it beforehand.
00:10:08 For instance, if we need to show various project details, we can design a single details page with a consistent layout for all projects.
00:10:17 The only difference is that the data or the content will change for each project.
00:10:23 So instead of making separate routes for every project details page, you can use a Next.js's dynamic route.
00:10:31 To create a dynamic route, we'll have to wrap the folder's name in square brackets, symbolizing that the content inside the square bracket is a variable.
00:10:40 So continuing on our current application, let's add a feature for displaying project details.
00:10:46 Imagine we have three projects, named jobit, current, and hipnode.
00:10:51 And coincidentally, these are the code names of the projects within the master class.
00:10:57 We need three routes to showcase these projects.
00:11:00 So if we go to our app and then our project list, we can now create three different folders.
00:11:06 JobIt, CarRent, and we can also do Hipnode.
00:11:13 And now we would have to create a new page.
00:11:16 Although the content is mostly going to be the same, we have to duplicate it instead of each one.
00:11:21 And imagine if you had 99 projects.
00:11:24 Or imagine if you allowed your users to add projects.
00:11:27 You would have the unlimited number of them.
00:11:29 So this is obviously not the optimal way.
00:11:32 And you would even have to keep copy pasting the similar project details code.
00:11:37 This is where dynamic routes come to rescue.
00:11:39 So let's delete all of these folders right here, and let me show you what I mean.
00:11:44 Within the existing projects folder, create a new folder with a name enclosed in square brackets.
00:11:51 So forward slash slug.
00:11:53 Slug is simply a name for the ID within the URL of that project.
00:11:57 But you can choose any name you want, such as ID or name.
00:12:02 In this case, let's use name for simplicity.
00:12:05 And within it, we can create a new page.tsx file.
00:12:10 For now, let's simply render what we did all the time, which is the project name.
00:12:15 To access this route, we will include a link to our hypothetical projects on the project's list page and give them a bit of styling.
00:12:24 So I'm going to import the styles.
00:12:26 And under my projects, I'm going to show a UL that's going to contain three different links.
00:12:34 So first, let's indent this properly.
00:12:37 And second, let's import the link coming from next link.
00:12:41 So pointing to project's job bit, project's current, and project's hip node.
00:12:46 And just to style it a bit, I can create this new projects.module.css and paste the styles right here.
00:12:56 Feel free to pause this video and type them out if you want to, but it's truly not necessary.
00:13:01 You can watch me go along.
00:13:03 And is there anything else that we need to do?
00:13:06 Well, no, that's it.
00:13:09 If you go back, you can see three of these links appear.
00:13:12 And if you click on them, sometimes it takes a bit longer on localhost, but on the deployed website, it is instantaneous.
00:13:20 and you will be redirected to projects in that URL and you'll be able to see project name.
00:13:25 Same thing, if we go back and click on current, you see you're on this link pointing to this page.
00:13:31 However, something doesn't feel good.
00:13:34 While the route for these projects changes correctly, it would be great to see the actual project name displayed on the respective routes page instead
00:13:43 of the static project name.
00:13:45 So, how do we do that?
00:13:47 Well, the name part here is our dynamic route segment, and Next.js provides us a way to access what value has been passed through the params prop passed
00:13:59 to the page.js.
00:14:01 So to utilize the value of this dynamic segment, we need to get it right here through params.
00:14:08 And then, that's going to include projectParams.name.
00:14:14 And this is going to be equal to whatever you have in the square brackets right here.
00:14:19 So if it was an ID, then you would have to say params.id.
00:14:22 Whatever name you provide, it will be the same name to access the value through the params object.
00:14:28 And now, if we go back, you'll see projectCurrent.
00:14:32 If we go to Home one more time and click See Projects and go to Jobit, you can see Project Jobit and Project Hypnote.
00:14:40 These are automatically created dynamic routes that we created by simply creating one simple dynamic route folder and file right here.
00:14:49 Pretty amazing, isn't it?
00:14:52 But that's not even the end of routing in Next.js.
00:14:56 Coming next, we have route groups.
00:15:00 While working in a file-based system, having many folders right here may not be ideal, because you can easily get lost within all of them.
00:15:09 But you have to have them, because that's how you do routing in XJS, right?
00:15:14 So to address this and offer better control over folder organization without impacting the URL structure, Next.js introduced a feature called route groups.
00:15:26 How do we make sense of it?
00:15:28 Well, consider the existing structure.
00:15:30 We already have three folders, about, contact, and projects.
00:15:35 Now, if we need to add more functionality like sign in and sign up, we would have to create more folders within the app folder,
00:15:42 causing it to grow larger and larger.
00:15:46 Well, what if we could limit the number of folders inside of the app folder to a maximum of 1 to 3 and include everything within these folders while maintaining
00:15:58 the same route path?
00:16:00 In this case, if we try to accomplish a structure that looks like this, where we have app and then auth and then sign in within it,
00:16:09 this is going to impact the routing.
00:16:12 Why?
00:16:12 Because as we learned, each folder name serves as a route.
00:16:17 So if we do it in this way, the route name for the sign in page would be forward slash auth, forward slash sign in.
00:16:25 And same thing for the sign up.
00:16:28 But we intended something else, right?
00:16:30 Our desired route names are simply sign in and sign up.
00:16:34 No auth beforehand.
00:16:36 But we still want to maintain a proper file organization.
00:16:39 We don't want the auth to be included in the URL, but we do want it in our current code structure.
00:16:46 To meet this specific requirement, we have route groups.
00:16:51 They help organize routes into logical groups like auth, team, dashboard, and more.
00:16:57 We can create a route group by enclosing the folder name in parentheses.
00:17:04 So let's create two different route groups within our application.
00:17:07 Primarily, we're going to do one called Auth and one called Dashboard.
00:17:15 But notice how I wrapped it in parentheses.
00:17:17 Now within it, we can create those Auth pages we're talking about.
00:17:21 So we're going to have a sign in and we're going to also have sign up.
00:17:28 And within those, we're going to have page.tsx that's going to say sign in and we can duplicate this and create a new page.tsx and this one is going to
00:17:44 say sign.
00:17:48 And now these are within the enclosed route group.
00:17:51 So here's how the structure appears.
00:17:54 Everything gets enclosed within here.
00:17:56 Now let's transfer the remaining folders about contact and projects into our dashboard route group.
00:18:02 So we'll simply drag and drop them within it.
00:18:11 Now, once you transfer all the files, a simple structure should look like this.
00:18:15 A simple auth folder with a sign in and sign up, and a simple dashboard folder with about, contact, and projects.
00:18:22 All set.
00:18:23 Now let's put our application to the test.
00:18:26 If we go to the homepage, everything works.
00:18:29 About.
00:18:30 Same thing.
00:18:32 Contact.
00:18:33 Works as well.
00:18:34 And then if you navigate to the project list, that works as well.
00:18:38 And each project details page works too.
00:18:42 How surreal.
00:18:44 No code breaks, and everything works flawlessly.
00:18:47 As if nothing had happened.
00:18:49 The URL, the linking, everything is functioning perfectly, and all on the first try.
00:18:55 Moreover, now if we modify a URL to sign in, you can see the sign in appear right here.
00:19:04 And same thing for the sign up.
00:19:06 It's not under auth sign in and the sign up, it's just sign in or sign up because we used route grouping.
00:19:14 That, my friend, is the beauty of Next.js 13. And is that the end?
00:19:20 End of routing?
00:19:21 Well, of course not.
00:19:23 There are two more amazing client-side routing features, parallel routes and intercepting routes.
00:19:29 And not to forget, we also have API routes.
00:19:33 We will discuss some more about these in the advanced routing chapter.
00:19:37 Now, I have a task for you.
00:19:40 Create a complete routing structure for an e-commerce project using different routes.
00:19:46 Here are the expected routes we want to have.
00:19:48 Homepage.
00:19:50 Product listing page.
00:19:51 product listing details page, shopping cart page, checkout page, order confirmation page, user account page, login page,
00:20:01 registration page, and search results page.
00:20:05 Try building it out using the route concepts we just learned.
00:20:10 Next, explain how does the Next.js routing differ from React.js routing?
00:20:16 What is the purpose of route groups and how can they be created in Next.js?
00:20:21 And finally, what is a dynamic route and why should we create dynamic routes in web applications?
00:20:28 This was a great lecture.
00:20:29 I hope it was truly eye-opening and I hope that you like this file-based routing system as much as I do.
00:20:36 At this point, I would highly recommend spinning up a new Next.js application and just playing with it a bit.
00:20:41 Try creating folders, files, route, pages, layouts and just test it all out.
00:20:47 See how it works under the hood.
00:20:49 You have to test it to be able to fully get it.
00:20:52 Not just listen to me talk.
00:20:54 So do some testing and I'll see you in the next lecture.