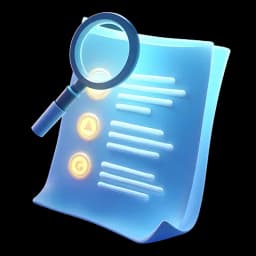
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
Complete source code available till this point of lesson is available at
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:02 Let's create the first and maybe the most important database model of our application.
00:00:07 It's going to be the user model.
00:00:09 And to make our application scalable, let's immediately start with a proper file and folder structure for our database models.
00:00:16 I'll create a new database folder at the root of our application.
00:00:20 And within it, I'll create a new file called user.model.ts.
00:00:26 Within it, we'll follow Mongoose's structure for creating our first model.
00:00:31 And I'm happy to say that as of recently, Mongoose officially supports TypeScript bindings within the index.ts file.
00:00:39 So that means that we can properly write TypeScript types, which will be consistent across our application, both on the front end and on the database side.
00:00:49 But again, let's not worry about this too much because I'll teach you how to do everything from scratch.
00:00:53 Let's start by defining a user schema.
00:00:57 We can do that by saying const user schema is equal to new schema coming from Mongoose.
00:01:03 We have to call it and then pass the schema definition.
00:01:06 Here, you can pass all the different fields that this specific model will have.
00:01:10 Like a user will have a name, it'll be of a type string and required to true.
00:01:16 It'll also have a user.
00:01:19 name equal to type string and required to true it'll also have an email of a type string required to true and they'll also pass it a unique property which
00:01:30 will be set to true that's going to look something like this what else does our user need to have well it's going to be a bio So some info about the user,
00:01:41 and we can make this type string, but it won't be required.
00:01:45 Let's also make sure that each user has an image of a type string, and required is set to true.
00:01:52 This will be a URL.
00:01:54 We can also ensure that each user has a location, like where they're from, like we can see on GitHub.
00:01:59 Maybe even a link to their portfolio, so they can display it.
00:02:03 and finally their reputation which is going to be of a type number and at the start it's going to be set to the default of zero.
00:02:12 After this we can also provide timestamps is set to true which will make sure to generate timestamps on when the user was created.
00:02:21 Once we have this user schema we can then define the user model by saying const user is equal to use the model functionality from Mongoose,
00:02:31 give the user a name like user, and then pass the user schema right into it.
00:02:37 And finally, export default this model of the user, which will then allow us to call the user dot create new document or something like that,
00:02:48 or even use the new user syntax that will allow us to then create a new user document with some properties.
00:02:55 But how can we optimize this even further?
00:02:58 Well, we don't want to create a new user model every time.
00:03:02 We just want to make sure to create it once.
00:03:04 So for that reason, on top of getting the model from Mongoose, we can also get access to models, which is going to give us access to all the currently
00:03:13 created models with this Mongoose instance.
00:03:16 So we can check if models?user already exists, then use that, else create a new model.
00:03:25 Now, this is going to be great for the backend.
00:03:28 It's going to tell us which fields do we need, but we want to make sure to accept proper TypeScript types so that our application is scalable and error-prone.
00:03:38 To do that, we can export a new interface called iUser, which stands for interface user, to differentiate it from user, which is the model.
00:03:49 So let's give it a name of a type string, username of a type string, email of a type string, bio of a type string, which will be optional.
00:04:00 image of a type string, and then location, portfolio, and reputation, which all three will be optional, with first two being strings and the reputation
00:04:10 being a number.
00:04:11 Now, we can define that type right here directly within the user model when we create it, by saying the model will be of a type or interface,
00:04:21 iUser.
00:04:23 This way, whenever you create a new model, on the front-end application, it'll know exactly which properties you have access to.
00:04:31 This is just beautiful, and it'll make our development workflow so much smoother.
00:04:36 Trust me on that.
00:04:37 And I even have to mention the amount of errors that it'll save us from, because if you type something like user.
00:04:45 Maybe user name like this, if you misspell it, it'll automatically point to the error saying that user name doesn't exist,
00:04:54 but user name does exist and it is of a type string.
00:04:59 So believe it or not, this is it.
00:05:01 This is our user model that soon enough we'll be able to use within our application.
00:05:06 There's nothing more to it.
00:05:07 This is how we create models in Mongoose and MongoDB.
00:05:10 Let's commit this by saying, create user model.
00:05:16 And commit and sync.
00:05:18 And now we can focus on adding all of the other models within our application.
00:05:22 But of course, we got to celebrate the small successes.
00:05:25 So congrats on creating your first database model.
00:05:28 Try to dissect it a bit first, look into it, think about it.
00:05:32 Try to understand why we're creating the interface and then why we're creating a Mongoose schema and then connecting the two to form a user model,
00:05:41 off of which we'll be able to create new type instances of that specific model.
00:05:47 Also, try to compare it with this diagram right here.
00:05:50 It's gonna be quite similar, but there are some differences that I have to fix, such as this password that we didn't add.
00:05:56 The portfolio website here says portfolio website and in the document just says portfolio and things like that.
00:06:02 But the most important part for me right now is that you understand that you're not just writing code right here, you're architecting what is yet to become
00:06:10 your future database structure.
00:06:12 And if you don't fully get it yet, that's totally okay.
00:06:15 We'll now repeat the same exact structure many, many times to create all of the other models for our application.
00:06:23 Great work.