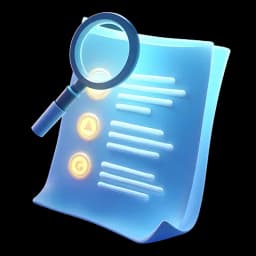
Quick Lecture Overview
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
Complete source code available till this point of lesson is available at
By logging in, you'll unlock full access to this and other free tutorials on JSM Pro.
Why? Logging in lets us personalize your learning experience, track your progress, and keep you in the loop with new workshops, coding tips, and platform updates.
You'll also be the first to know about upcoming launches, events, and exclusive discounts.
No spam—just helpful content to level up your skills.
If that sounds fair, go ahead and log in to continue →
Enter your name and email to get instant access
##Looks like we found a thief monkey By the way, I liked the trick how you reached till here. You have a good sense of humor. You will improve a lot if you join our course with this passion.
var
(function-scoped, outdated)let
(block-scoped, modern and recommended)const
(block-scoped, cannot be reassigned)_
, or $
let let = 5;
is invalid)myVar
and myvar
are different)string
, number
, boolean
, null
, undefined
, bigint
, symbol
Objects
, Arrays
, Functions
Subscribing gives you access to a brief, insightful summary of each lecture to stay on track.
00:00:02 After the dynamic user routes, I have another route which I want to add, but it's not going to be dynamic.
00:00:09 It's going to be users forward slash or rather new folder, email.
00:00:15 And within the email, we can create a new route.ts.
00:00:20 Now, why do we need an email route and what does that even mean?
00:00:24 Well, sometimes we might want to get a user by its email and not ID.
00:00:30 For that reason, we can create this new API route.
00:00:33 We'll specifically use this within the Auth where we want to see if a user email exists.
00:00:38 And if so, then we can get all of the user-associated accounts.
00:00:43 So the process is going to be similar as find by ID, but just a tiny bit different.
00:00:48 Let me explain how it works.
00:00:49 It's going to be an export async function.
00:00:52 And believe it or not, it's going to be post and not get.
00:00:57 So here we're going to get the request.
00:01:00 of a type request.
00:01:02 And the reason why it's post is because we're going to extract the email right here from the body.
00:01:08 So we can say is equal to await request.json.
00:01:12 We can open up a typical try and catch block.
00:01:16 In the error, we can just return a handle error coming from lib handlers.
00:01:21 To it, we can pass the error.
00:01:23 We can say that it is an API error.
00:01:25 And we can say as API error response.
00:01:29 In the try, we can try to validate this data by saying const validated data is equal to user schema dot partial, because we're only passing partial data,
00:01:43 dot safe parse.
00:01:46 And to it, we want to pass an object containing the email field.
00:01:51 If it's not a valid email, so if not validateddata.success, in that case, we can simply throw a new validation error and we can pass validateddata.error.flatten
00:02:13 and then dot field errors.
00:02:16 This way we'll know exactly what we have to fix.
00:02:18 Finally, if we do have the email, we can try to get the user by saying const user is equal to await user, which is the user schema or model,
00:02:31 dot find one.
00:02:33 And in this case, we're not finding by ID, we're finding by email.
00:02:38 If a user doesn't exist, so if there is no user, we can throw a new not found error coming from lib errors, and we can say user,
00:02:49 because what is not found, the user is not found.
00:02:53 Finally, if we do get the user, we can return next response.
00:03:02 where success will be set to true, data will be set to user, and finally, the status will be set to 200. And now we can try to get this user by email.
00:03:13 I'll check it with my Thunder client by trying to make a post request.
00:03:18 And let's try to remember this email, JohnDoe at gmail.com.
00:03:22 So I'll actually copy this entire line.
00:03:24 We'll make that request to users email.
00:03:28 And in the JSON body, we actually have to pass the email which we want to find.
00:03:33 So that's going to look something like this.
00:03:36 Email is John Doe.
00:03:38 And if I try to send it, we get a 200 and we get back that user, which is exactly what we wanted.
00:03:44 Great, this was a pretty simple lesson, but again, it just shows you how we can create all of these different routes, and we're just following a structure.
00:03:53 We find a specific route, which we want to expose on the backend side, so we can call it from the frontend.
00:04:00 Then we export a new async function with the code name of the HTTP request, which we want to use when we call it.
00:04:07 Then we extract either the query params, the regular params, or the request.
00:04:12 do some logic, most often it's going to be just a database connection or mutation, and then return the necessary data to the frontend.
00:04:19 That's it.
00:04:20 So let me also say implement.
00:04:24 user by email, commit, sync, and we are ready to add some more route handlers.
00:04:31 Now, we are moving from user routes to account routes.
00:04:35 Remember, a user can have multiple accounts, and each account is for one provider, such as email, Google, or GitHub, or other social accounts.